1. 이벤트 핸들러 선언
이벤트 핸들러 대리자를 전역으로 선언한다. 가급적이면 이벤트가 실제 발생하는 위치에 선언하는 것이 좋다.
2. 이벤트 등록
이벤트가 발생할 클래스 내부에 위에서 선언한 이벤트 핸들러 대리자로 이벤트 변수를 정의한다.
이벤트 += 이벤트처리함수
3. 이벤트 해제
이벤트를 해제한 후에는 이벤트가 발생해도 이벤트처리함수가 작동하지 않는다
이벤트 -= 이벤트처리함수
4. 사용 예제
1) 이벤트가 실제 발생하는 곳
using System;
using System.Collections.Generic;
using System.ComponentModel;
using System.Data;
using System.Diagnostics;
using System.Drawing;
using System.Linq;
using System.Text;
using Systehttp://m.Threading.Tasks;
using Systehttp://m.Windows.Forms;
namespace WindowsFormsApp2
{
/// <summary>
/// 마우스 다운 핸들러 선언
/// </summary>
/// <param name="sender"></param>
/// <param name="e"></param>
public delegate void DgvMouseDownEventHandler(object sender, EventArgs e);
public partial class MyDataList : UserControl
{
#region 이벤트 등록
/// <summary>
/// 마우스 다운 이벤트
/// </summary>
public event DgvMouseDownEventHandler DgvMouseDown;
#endregion
#region Attributes
[Browsable(true)]
[Category("MyDataList")]
[Description("데이터목록의 열집합")]
public DataGridViewColumnCollection Columns
{
get { return dgvDatas.Columns; }
set
{
if (value != null && value.Count > 0)
{
foreach (DataGridViewColumn v in value)
{
dgvDatas.Columns.Add(v);
}
}
}
}
[Browsable(false)]
[Category("MyDataList")]
[Description("데이터목록의 행집합")]
public DataGridViewRowCollection Rows
{
get { return dgvDatas.Rows; }
}
/// <summary>
/// 행번호를 자동으로 생성할지 여부 (default=false). RowHeadersVisible=true일 때 사용 가능함
/// </summary>
private bool m_bAutoIndex = false;
[Browsable(true)]
[Category("MyDataList")]
[Description("행번호를 자동으로 생성할지 여부. RowHeadersVisible=true일 때 사용 가능함")]
[DefaultValue(false)]
public bool IsAutoIndex
{
get { return m_bAutoIndex; }
set
{
m_bAutoIndex = value;
}
}
/// <summary>
/// 자동으로 생성되는 행의 시작번호 (default=1)
/// </summary>
private int m_nAutoIndexStartNo = 1;
[Browsable(true)]
[Category("MyDataList")]
[Description("자동으로 생성되는 행의 시작번호")]
[DefaultValue(1)]
public int AutoIndexStartNo
{
get { return m_nAutoIndexStartNo; }
set
{
if (value < 0)
return;
m_nAutoIndexStartNo = value;
}
}
#endregion
#region 생성자
/// <summary>
/// 생성자
/// </summary>
public MyDataList()
{
InitializeComponent();
}
#endregion
#region 컨트롤 이벤트 처리
/// <summary>
/// 컨트롤이 화면에 표시되기 직전 이벤트
/// </summary>
/// <param name="sender"></param>
/// <param name="e"></param>
private void MyDataList_Load(object sender, EventArgs e)
{
dgvDatas.RowHeadersVisible = IsAutoIndex;
}
private void dgvDatas_MouseDown(object sender, MouseEventArgs e)
{
Debug.WriteLine($"dgvDatas_MouseDown: {e.Button}, {e.Location}");
if (e.Button == MouseButtons.Left)
{
if (DgvMouseDown != null)
{
DgvMouseEventArgs args = new DgvMouseEventArgs();
args.Pos = e.Location;
args.Button = e.Button;
var ht = dgvDatas.HitTest(e.X, e.Y);
if (ht != null)
{
args.Col = ht.ColumnIndex;
args.Row = ht.RowIndex;
Debug.WriteLine($"dgvDatas_MouseDown: {e.Button}, {e.Location}, {args.Row}, {args.Col}");
}
DgvMouseDown(dgvDatas, args);
}
}
}
/// <summary>
/// DataGridView 의 RowHeader 에 일련번호 자동생성
/// </summary>
/// <param name="sender">DataGridView 컨트롤</param>
/// <param name="e">이벤트정보</param>
/// <param name="startno">시작번호</param>
/// <remarks>DataGridView의 RowPostPaint 이벤트에 사용함</remarks>
public void DGV_AutoRowIndex(object sender, DataGridViewRowPostPaintEventArgs e)
{
if (IsAutoIndex && sender is DataGridView)
{
try
{
DataGridView dgv = sender as DataGridView;
if (dgv != null && dgv.RowHeadersVisible && dgv.Rows.Count > 0 && e.RowIndex >= 0)
{
String rowIdx = (AutoIndexStartNo + e.RowIndex).ToString();
StringFormat centerFormat = new StringFormat()
{
Alignment = StringAlignment.Center,
LineAlignment = StringAlignment.Center
};
RectangleF headerBounds = new RectangleF(e.RowBounds.Left, e.RowBounds.Top, dgv.RowHeadersWidth, e.RowBounds.Height);
e.Graphics.DrawString(rowIdx, dgv.Font, SystemBrushes.ControlText, headerBounds, centerFormat);
}
}
catch (Exception ex)
{
Debug.WriteLine(ex.ToString());
}
}
}
#endregion
}
#region DataGridView 마우스이벤트 매개변수
/// <summary>
/// DataGridView 마우스이벤트 매개변수 클래스
/// </summary>
public class DgvMouseEventArgs : EventArgs
{
/// <summary>
/// 마우스 클릭시 사용된 버튼
/// </summary>
public MouseButtons Button { get; set; }
/// <summary>
/// 마우스 클릭시 커서의 위치
/// </summary>
public Point Pos { get; set; }
/// <summary>
/// 마우스 클릭시 DataGridView의 열번호
/// </summary>
public int Col { get; set; }
/// <summary>
/// 마우스 클릭시 DataGridView의 행번호
/// </summary>
public int Row { get; set; }
/// <summary>
/// 생성자
/// </summary>
public DgvMouseEventArgs() { }
}
#endregion
}
2) 이벤트를 사용할 곳
using System;
using System.Collections.Generic;
using System.ComponentModel;
using System.Data;
using System.Diagnostics;
using System.Drawing;
using System.Linq;
using System.Text;
using Systehttp://m.Threading.Tasks;
using Systehttp://m.Windows.Forms;
namespace WindowsFormsApp2
{
public partial class Form1 : Form
{
public Form1()
{
InitializeComponent();
}
private void myDataList1_DgvMouseDown(object sender, EventArgs e)
{
DgvMouseEventArgs args = (DgvMouseEventArgs)e;
Debug.WriteLine($"dgvDatas_MouseDown: {args.Button}, {args.Pos}, {args.Row}, {args.Col}");
}
}
}
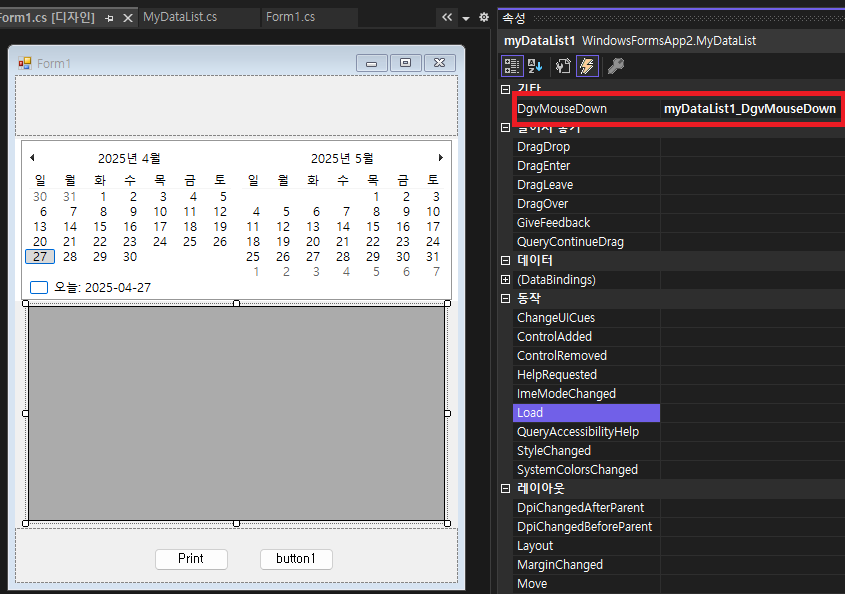
'유용한 정보' 카테고리의 다른 글
[clr] Window Forms 컨트롤 라이브러리 MFC에서 사용하기 사용 (0) | 2025.05.06 |
---|---|
[C#] DataGridView 자동 행 번호 생성하는 방법(예제) (0) | 2025.05.05 |
[C#] MonthCalendar 요일 클릭하기 (0) | 2025.04.24 |
Windows 10에서 Visual Studio 6(vc6.0) 설치 방법 (2) | 2025.04.22 |
[MFC] 윈도우 레지스트리의 키 또는 데이터를 읽고 쓰고 삭제하기 (0) | 2025.04.19 |